[EthersJs - 3] What's you address bro!
In the end, blockchain is a trustless distributed ledger that keeps a running tally of transactions between addresses.
In the case of Ethereum, there are two kinds of addresses:
- Externally Owned account - aka individuals with private keys
- Smart Contract accounts - addresses governed by code.
Therefore, to do anything, we must learn how to generate and import addresses with Ethers JS.
Let's start by creating an Ethereum address. Let's copy paste the following code in play.js
and then understand how the snippet works.
const ethers = require("ethers");
const crypto = require('crypto')
// Step 1: generate a private key
const id = crypto.randomBytes(32).toString('hex')
const privateKey = "0x"+id;
console.log("Generated Private Key", privateKey)
// Step 2: generate wallet from the private key
const wallet = new ethers.Wallet(privateKey)
console.log("Generated Wallet Address", wallet.address)
First, we import ethers and crypto module.
Then, in Step 1, we generate a private key. We know that the ethereum private keys are 64 random hex characters or 32 random bytes. So, we generate that with the command crypto.randomBytes(32).toString('hex')
Then, we just add the prefix 0x
to it.
In Step 2, we use Ethers' Wallet Api to generate a wallet based on the private key we generate in Step 1.
const wallet = new ethers.Wallet(privateKey)
Running the Script with node play
we get:
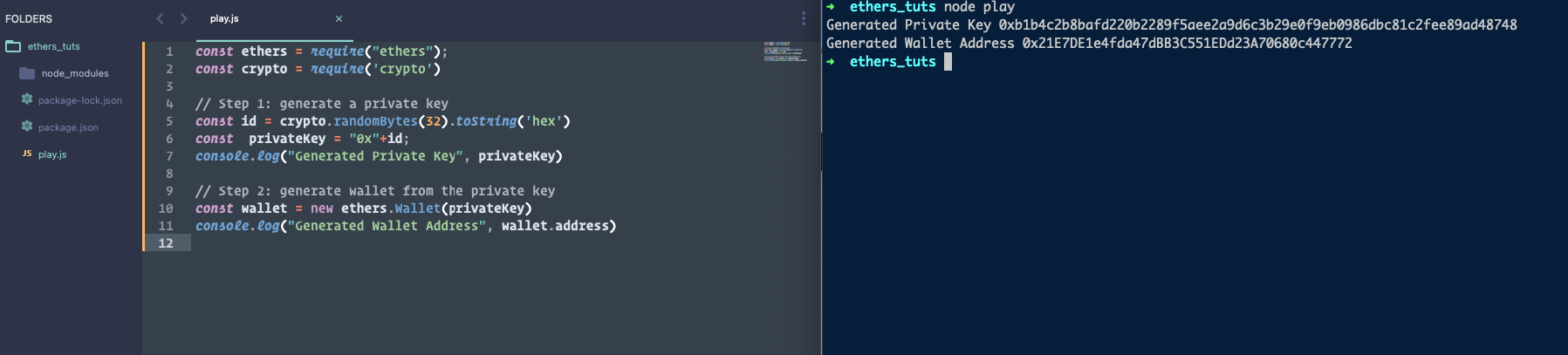
And that is it! you created your first Ethereum address using Ethers JS
Note: You should save the private key if you plan on using this address, and never share the private key with anyone else.
If you already have a private key, and want to use that address, you can skip Step 1, and go to Step 2 directly.
If you use Metamask, you can get the private key quite easily by hitting the three vertical dotted lines and then, going to the account details.
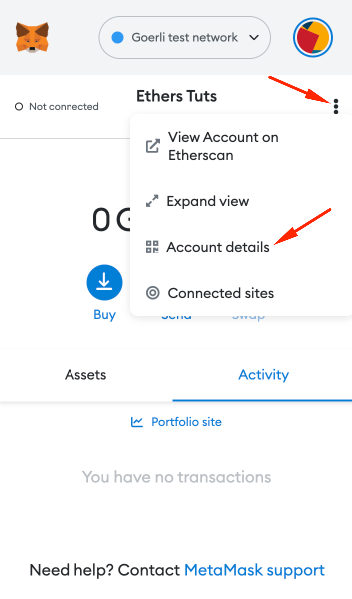
And then, Exporting the private key from the details page
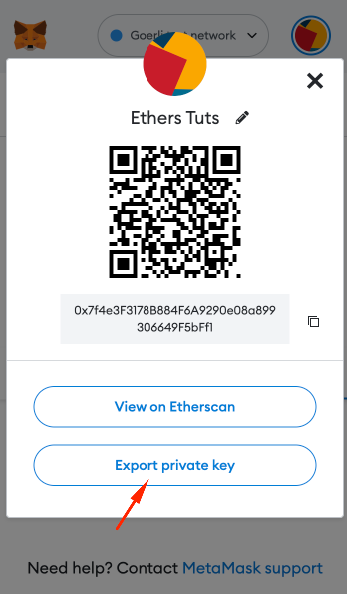
Note: At the risk of sounding like a broken record, anyone with access to your private keys, has access to everything in your account. DO NOT share this key with anyone, take care while using the key to write code, as you do not want this to end on in a public repository.
Do not use your main account for this tutorial, make a new account which does not have any money to learn.
With access to private key, our code will look like:
const ethers = require("ethers");
const privateKey = "0xe653b96c34dfb3887fc8b29d68655fd57d7cd4dc3d34f4988893fb0c6f6c6047"
const wallet = new ethers.Wallet(privateKey)
console.log("Generated Wallet Address", wallet.address)
So, now we know how to create and import addresses using Ethers JS. Let's learn a few more tricks you can perform with ethers to make your life easier.
Creating Random Wallets with one command:
Random wallets are so commonly created in Ethers, that ethers gives us a easy way to do it - Wallet.createRandom()
const ethers = require("ethers");
// creating random wallet
const walletRandom = ethers.Wallet.createRandom()
console.log("Random Wallet address", walletRandom.address)
console.log("Random wallet private key", walletRandom.privateKey)
console.log("Random wallet mnemonic", walletRandom.mnemonic)
In this case, we will not be needing the crypto module to generate private key. Ethers abstracts away all that work for us.
Once we have instantiated the wallet object, we have access to various properties to make our lives easier:
wallet.address
gives us the addresswallet.privateKey
gives us the private key for the generated wallet.- We can even get the mnemonic with
wallet.mnemonic
Running the new script gives us:
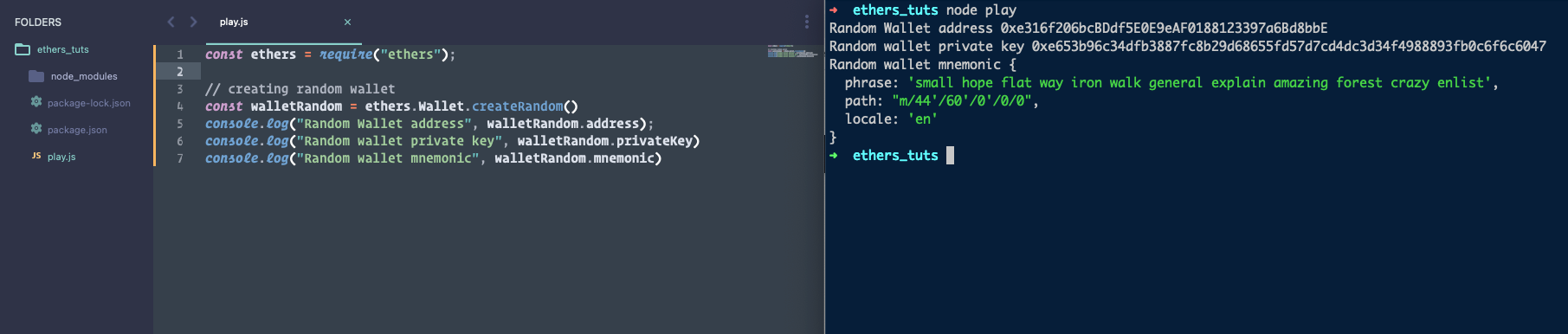
Now you can create and import addresses using ethers JS easily!
Ethers Js also lets us import wallets using mnemonic using the command - Wallet.fromMnemonic([ypur-mnemonic-here])
We can use it like so:
const ethers = require("ethers");
const mnemonic = "story mirror type myself busy egg cereal dolphin taste sister exclude pudding"
const walletRandom = ethers.Wallet.fromMnemonic(mnemonic)
console.log("Random Wallet address", walletRandom.address);
console.log("Random wallet private key", walletRandom.privateKey)
So, in summary, we learned:
- How to generate random private key and generate wallet based on the generated private key.
- How to generate random wallet very easily using just ethers Js in one step and access various properties of the wallet
- Importing wallets easily with private key or mnemonic